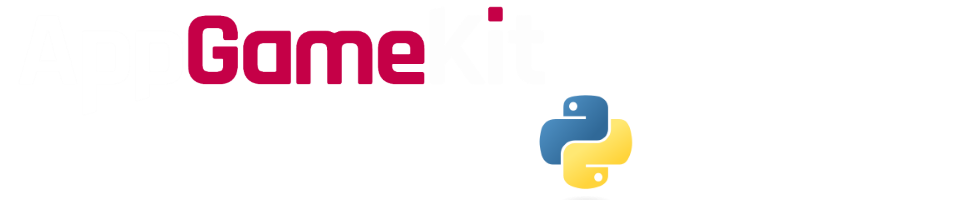
AppGameKit for Python
A downloadable tool for Windows and Linux
Welcome to the world of game development using AppGameKit for Python!
AppGameKit for Python is a Python 3 C extension module of a complete, full-featured implementation of AppGameKit Classic for Windows (both 32- and 64-bit) and Linux (64-bit). Included is an HTML command reference as well as a PYI file to help IDEs, such as PyCharm, understand the command implementation and provide contextual help. Since AppGameKit for Python is free for use, AppGameKit is advertised by being mentioned in the window title bar and by the AppGameKit logo for a few seconds on start-up.
If you find this project useful, please buy AppGameKit (if you haven't already). You can also save 50% off AppGameKit and any of the bundles by using this promo code during checkout: AGKPYTHONDEAL50. Feel free to let The Game Creators know that you're liking AppGameKit for Python. :)
Thanks for using AppGameKit for Python! If you have any questions or problems, feel free to create a topic in the community forum.
Under the current license I can’t charge money for this project, but I am allowed to accept donations and they will be greatly appreciated.
Installation and Usage
Using this extension module is as easy as adding the "appgamekit" folder found in the ZIP download below into your project folder and importing. If you use an IDE such as PyCharm, you can add the appgamekit.pyi file to your project folder as well so the IDE can better understand the extension module's contents.
Once this is done, you're on your way to developing great games with AppGameKit for Python!
import appgamekit as agk with agk.Application(): while True: agk.print_value("Hello, World!") agk.sync() if agk.get_raw_key_pressed(agk.KEY_ESCAPE): break
Downloadable Content
The AppGameKit asset downloadable content packs will work with the Python version and are worth checking out:
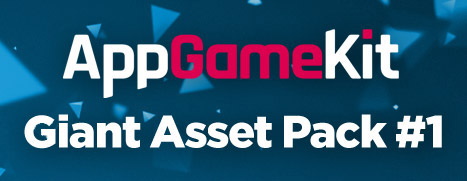
Differences from AppGameKit Tiers 1 and 2
Method Names
Method names have been changed so that they follow the PEP 8 style guide. The AppGameKit methods in Tiers 1 and 2 are TitleCased, but for this they are lowercase_with_underscores. ie: The LoadImage method is called load_image here.
The Print method was renamed to print_value so that it wouldn't be confused with Python's builtin print command.
Method Overloads
Since Python doesn't have method overload like C++ and AppGameKit Basic, some method names have been modified to compensate. An example of method overloading can be seen with the LoadImage command which has four method overloads:
LoadImage( ID, sImageFilename ) LoadImage( ID, sImageFilename, bBlackToAlpha ) integer LoadImage( sImageFilename, bBlackToAlpha ) integer LoadImage( sImageFilename )
The first two take an arbitrary ID that you provide as the first parameter and the last two return the ID that AppGameKit assigns internally instead. There's also a bBlackToAlpha parameter appearing in one of the overloads of each set.
For AppGameKit for Python, these two sets are combined into two methods, each with an optional parameter.
load_image(filename, black_to_alpha=False) load_image_id(image_id, filename, black_to_alpha=False)
And the AppGameKit Tiers 1 and 2 code for:
LoadImage(1, "img.png")
becomes this in AppGameKit for Python:
load_image_id(1, "img.png")
Read Path
AppGameKit builds its internal "read" path based upon the main process executable name. For Python scripts, the read path for every script is based on the Python interpreter which means every script has the same read path.
This obviously is not desirable, so AppGameKit for Python monitors all methods requesting to open files and changes paths that are relative to the read path so that they are absolute ("raw") paths based upon where the main script is being executed.
This change allows you to be able to use a folder structure like that of AppGameKit Tiers 1 and 2 in that the "media" subfolder will be located in the same folder as your main script file.
Plugins
AppGameKit for Python supports loading Tier 1 plugins with its import_plugin method.
plugin = import_plugin("ExamplePlugin") print(plugin.AddFloat(5, 7)) # prints 12.0
In order to use a plugin, its folder will need to be copied into a subfolder called "Plugins" where your main script is located. The resulting file structure will be something like this:
main.py appgamekit / __init__.py _x64 / appgamekit.pyd appgamekit.so _x86 / appgamekit.pyd Plugins / ExamplePlugin / Commands.txt Windows.dll
Unlike Tier 1, either the Commands.txt file for the plugin also needs to be in the plugin folder and distributed with your game or passed as a string when calling import_plugin. Plugin method names will have the same character casing specified in the Commands.txt file.
Multiple command definitions with the same command name in the Commands.txt file will be loaded, however only the last definition processed will be available. In order to have access to all command definitions, the Commands.txt file will need to be changed such that each command definition has a unique name.
Additions
The Application class encapsulates the creation and destruction of the AppGameKit window. It is recommended that developers instantiate an Application object using a with block and put all game code inside the with block.
The enable_debug_log() method has been added to turn on log() method output. Without calling this first, log() calls do nothing. This allows one to enable the log() method calls during development and leave them disabled for release easily with a single command call.
The extension module also contains several constants (such as key codes, alignment settings, etc) that can be used. They are listed at the top of the PYI file.
Exclusions
- Any method listed as being deprecated or not functioning on the AppGameKit help site. This includes the non-OGG music methods.
- PrintC was merged into the print_value method as the newline argument. When newline is True, the default, Print is used internally. When newline is False, PrintC is used instead.
- Str, Bin, Hex, Val, ValFloat, Left, Right, Mid, Asc, Len, Chr, Upper, Lower, Spaces, Round, Abs, Mod, and Pow. Python already includes these functions in some form.
- The Log math method creates ambiguity with the Log debug command. Use math.log() instead.
Notes
- This module can be used with PyInstaller in both "one file" and "one folder" bundles. The read path will be based upon the application location.
- It is not possible to change the taskbar icon when running from a Python interpreter. Windows uses the icon from the executable for the taskbar. If you use PyInstaller, you can set the EXE icon for your distribution.
- The print_value() and log() methods can handle any Python object. They use the __str__ representation of the object for the text they output.
End User License Agreement
In addition to AppGameKit's EULA:
- The end user is permitted to create any type of project with this extension module, freeware or commercial, even in the event that this project is removed from distribution.
- The end user agrees not to distribute this module except as part of their game's distribution package.
Copyright and License
AppGameKit and the AppGameKit Logo are copyright The Game Creators Ltd. All Rights Reserved.
This Python version of AppGameKit is officially licensed by The Game Creators Ltd to Adam Biser.
Windows, Mac, and Linux versions of AppGameKit are available from The Game Creators HERE.
The Python logo is a trademark of the Python Software Foundation.
Status | Released |
Category | Tool |
Platforms | Windows, Linux |
Rating | Rated 5.0 out of 5 stars (7 total ratings) |
Author | Fascimania |
Made with | AppGameKit |
Tags | gamedev, Game engine, python |
Links | Homepage |
Download
Click download now to get access to the following files:
Development log
- Version 2022.09.28Oct 27, 2022
- Version 2022.06.27Jul 21, 2022
- Version 2021.06.14Jun 21, 2021
- Version 2021.02.10 rev 1Mar 15, 2021
- Version 2021.02.10Mar 10, 2021
- Version 2020.11.16Dec 10, 2020
- Version 2020.04.30 rev 1Aug 03, 2020
- Version 2020.04.30May 08, 2020